浮动菜单
Floating Menu
此扩展程序将使菜单出现在空行中。
npm install @tiptap/extension-floating-menu
用法示例
基础
🌰 举个例子
可编辑
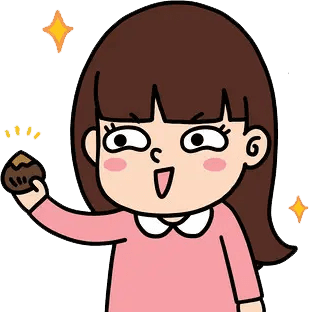
import { FloatingMenu, EditorContent, useEditor } from "@tiptap/react";
import StarterKit from "@tiptap/starter-kit";
import React, { useEffect } from "react";
export default () => {
const editor = useEditor({
extensions: [StarterKit],
content: `
<p>
输入新行(换行),将显示一些按钮。
</p>
<p></p>
`,
});
const [isEditable, setIsEditable] = React.useState(true);
useEffect(() => {
if (editor) {
editor.setEditable(isEditable);
}
}, [isEditable, editor]);
return (
<>
<div>
<input
type="checkbox"
checked={isEditable}
onChange={() => setIsEditable(!isEditable)}
/>
可编辑
</div>
{editor && (
<FloatingMenu editor={editor}>
<button
onClick={() =>
editor.chain().focus().toggleHeading({ level: 1 }).run()
}
className={
editor.isActive("heading", { level: 1 }) ? "is-active" : ""
}
>
标题1
</button>
<button
onClick={() =>
editor.chain().focus().toggleHeading({ level: 2 }).run()
}
className={
editor.isActive("heading", { level: 2 }) ? "is-active" : ""
}
>
标题2
</button>
<button
onClick={() => editor.chain().focus().toggleBulletList().run()}
className={editor.isActive("bulletList") ? "is-active" : ""}
>
无须列表
</button>
</FloatingMenu>
)}
<EditorContent editor={editor} />
</>
);
};
自定义显示逻辑
🌰 举个例子
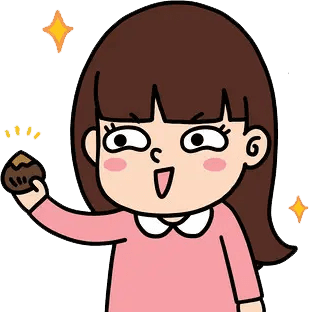
import { BubbleMenu, EditorContent, useEditor } from "@tiptap/react";
import StarterKit from "@tiptap/starter-kit";
import React from "react";
export default () => {
const editor = useEditor({
extensions: [StarterKit],
content: `
<p>
输入新行(换行),将显示一些按钮。
</p>
<p></p>
`,
});
const shouldShow = ({ editor, view, state, oldState, from, to }) => {
// 任何段落均会展示浮动菜单
return editor.isActive("paragraph");
};
return (
<>
{editor && (
<BubbleMenu editor={editor} shouldShow={shouldShow}>
<button
onClick={() =>
editor.chain().focus().toggleHeading({ level: 1 }).run()
}
className={
editor.isActive("heading", { level: 1 }) ? "is-active" : ""
}
>
标题1
</button>
</BubbleMenu>
)}
<EditorContent editor={editor} />
</>
);
};
API
属性 | 说明 | 类型 | 默认值 |
---|---|---|---|
tippyOptions | 本扩展基于tippy.js,此参数(如延迟显示等)将完全传递给它 | Object | |
shouldShow | 自定义显示菜单的逻辑 | (props) => boolean | - |
updateDelay | 更新延迟 | Number | 250 毫秒 |
element | 菜单挂载点(原生使用方式会用到) | HTMLElement | null |
pluginKey | 扩展唯一标识(一般用不到) | string | PluginKey |