加粗
Bold
使用此扩展以粗体呈现文本。
如果您在编辑器的初始内容中有<strong>
标签或带有相关的内联样式的,它们都会相应地呈现。
npm install @tiptap/extension-bold
用法示例
基础
🌰 举个例子
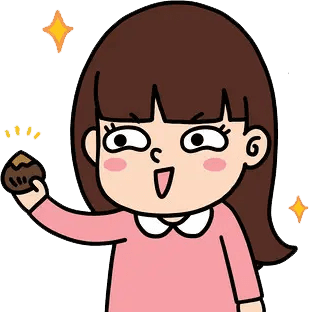
import Bold from '@tiptap/extension-bold'
import Document from '@tiptap/extension-document'
import Paragraph from '@tiptap/extension-paragraph'
import Text from '@tiptap/extension-text'
import { EditorContent, useEditor } from '@tiptap/react'
import React from 'react'
export default () => {
const editor = useEditor({
extensions: [Document, Paragraph, Text, Bold],
content: `
<p>这不是粗体。</p>
<p><strong>这是粗体。</strong></p>
<p><b>还有这个也是。</b></p>
<p style="font-weight: bold">这依然是.</p>
<p style="font-weight: bolder">哦, 还有这行!</p>
<p style="font-weight: 500">酷, 这难道不是吗!?</p>
<p style="font-weight: 999">粗到飞起的999!!!</p>
`,
})
if (!editor) {
return null
}
return (
<>
<button
onClick={() => editor.chain().focus().toggleBold().run()}
className={editor.isActive('bold') ? 'is-active' : ''}
>
粗体切换
</button>
<button
onClick={() => editor.chain().focus().setBold().run()}
disabled={editor.isActive('bold')}
>
设置粗体
</button>
<button
onClick={() => editor.chain().focus().unsetBold().run()}
disabled={!editor.isActive('bold')}
>
取消粗体
</button>
<EditorContent editor={editor} />
</>
)
}
API
方法 | 说明 |
---|---|
setBold | 将文本标记为粗体 |
toggleBold | 切换粗体标记 |
unsetBold | 删除粗体标记 |
举个例子:
editor.commands.setBold()